If you are just getting started with Python, one of the common errors you will come through is the List Index Out Of Range python error.
In this article, we will exactly see the cause of this error and how you can solve it, or to put it in other words, how you can avoid it. But before we get to the actual solution, let’s understand what the error is.
What is List Index Out of Range error in Python?
The List Index Out of Range Error in Python occurs if an invalid indices is accessed in your Python list. For example, if there is a list with 100 elements and your list contains only 5 elements, python will throw an error stating the List Index is out of range.
Here’s an example:
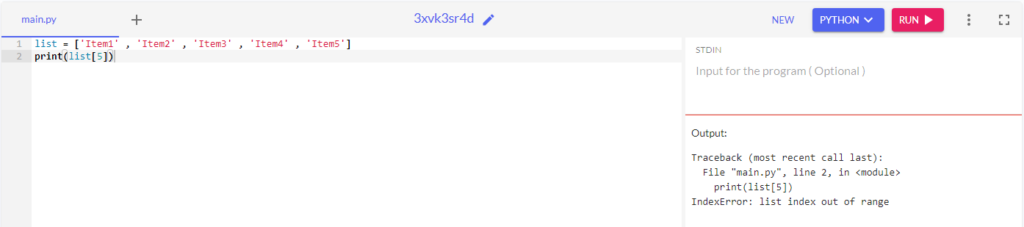
list = ['Item1' , 'Item2' , 'Item3' , 'Item4' , 'Item5']
print(list[5])
In our example, we are trying to print the Item 5 from our list and according to the output, it doesn’t exist. According to the code above, we have created a list and we are trying to print the 5th item from the list. But the python compiler outputs IndexError: list index out of range.
Wondering why?
This is a very simple and common mistake that everyone makes. In Python, the first element starts with 0 and not 1. So according to our item list –
list[0] – Item1
list[1] – Item2
list[2] – Item3
list[3] – Item4
list[4] – Item5
Our range starts from 0 (Item1) to 4 (Item5) thereby when we try to print(list[5]), automatically the compiler will throw the IndexError: list index out of range.
Now that you know this error occurs due to the range, you can easily find the range of your list with a simple line of code.
How to find the range in Python?
The range() function n Python is yet another useful function built-in Python that prints the list of sequences starting from zero to the number of items present in the range.
Here’s the additional line of code that we added in our example to see the range :
list = ['Item1' , 'Item2' , 'Item3' , 'Item4' , 'Item5']
for list in range(len(list)):
print(list)
Output:
0
1
2
3
4
In our example, list is the parameter we have used to implement the range loop and we have used the len function to list down the range of your list parameter.
So, when we execute the command for list in range(len(list)): with our existing line of code, we get the output 0,1,2,3,4 which is the same range we had discussed earlier –
list[0] – Item1
list[1] – Item2
list[2] – Item3
list[3] – Item4
list[4] – Item5
This simple line of code can be used to find the range in your python code and you can modify your print statement accordingly.
Now that you know how to find the range in a given code, let’s see how we can fix the IndexError : list index out of range error in python.
How to fix the IndexError : list index out of range error in Python?
So getting back to our code, we are trying to print the item5 from the list we just created –
list = ['Item1' , 'Item2' , 'Item3' , 'Item4' , 'Item5']
print(list[5])
Output:
Traceback (most recent call last):
File "main.py", line 2, in <module>
print(list[5])
IndexError: list index out of range
This can be fixed in two ways:
Method 1: Adding an extra item in your range
As mentioned earlier, in our example list, we had the range from 0 to 4. We can add an additional item to the list as the range 5 is not present in the code. This can be easily done by adding an additional item to your list (Item6). And here’s what it looks like –
list = ['Item1' , 'Item2' , 'Item3' , 'Item4' , 'Item5' , 'Item6']
print(list[5])
Output:
Item6
Method 2: Print item from the correct range
If you are trying to print Item5 from the list, then we have to print the correct item from the range. This can be done by changing our print from print(list[5]) to print(list[4]) and this should print the 4th item in your list which is Item5.
list = ['Item1' , 'Item2' , 'Item3' , 'Item4' , 'Item5']
print(list[4])
Output:
Item5
Conclusion
Python is all about trial and error.
The index out of range is a pretty simple error that most of us make, and as you guessed it right, the solution is pretty simple as well.
Hopefully, we have helped you solve the IndexError : list index out of range error along with a live example and different ways to solve it.
Also Read:
7 Best sites to learn Javascript online with Certificate 2022